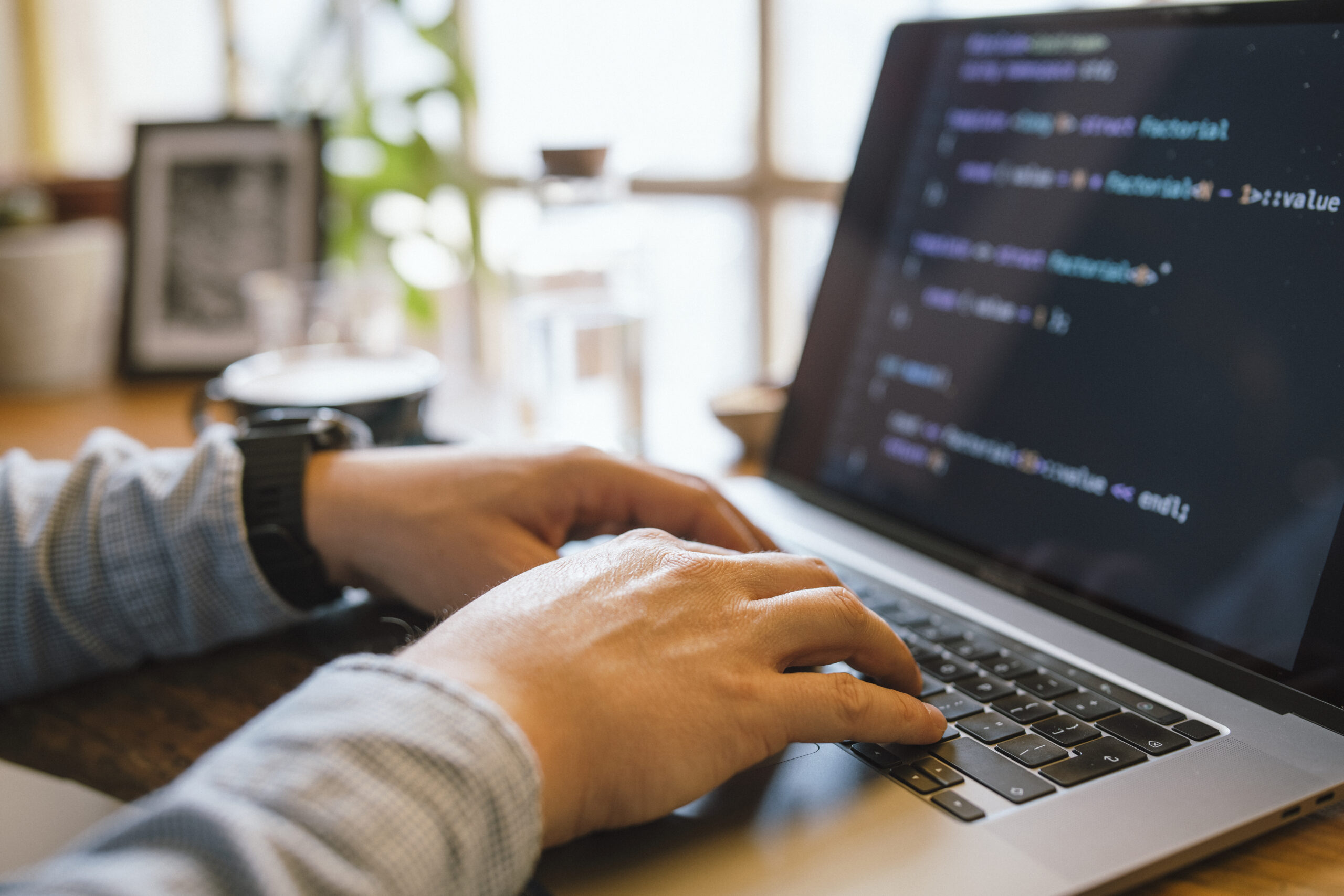
Debugging is Probably the most vital — still often ignored — expertise in the developer’s toolkit. It's actually not pretty much fixing broken code; it’s about knowing how and why factors go Erroneous, and Discovering to Imagine methodically to solve difficulties effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can help save several hours of annoyance and considerably transform your productiveness. Allow me to share quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use every single day. Even though creating code is one Element of progress, being aware of tips on how to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments occur Outfitted with potent debugging abilities — but several builders only scratch the area of what these resources can perform.
Just take, as an example, an Built-in Advancement Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code about the fly. When used effectively, they Allow you to notice specifically how your code behaves all through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They enable you to inspect the DOM, monitor network requests, watch real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can change discouraging UI problems into manageable jobs.
For backend or system-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Regulate over working processes and memory management. Finding out these instruments may have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code record, uncover the precise minute bugs were being released, and isolate problematic changes.
Ultimately, mastering your instruments usually means likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your growth natural environment making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you realize your resources, the more time you can spend solving the particular trouble instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently neglected — ways in helpful debugging is reproducing the situation. In advance of jumping into the code or making guesses, builders have to have to make a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code adjustments.
Step one in reproducing an issue is accumulating as much context as possible. Check with queries like: What steps led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have, the much easier it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve gathered sufficient information and facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not merely enable expose the issue and also reduce regressions Later on.
Often, The difficulty might be ecosystem-particular — it would transpire only on certain operating techniques, browsers, or underneath certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the condition isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging instruments much more efficiently, check prospective fixes securely, and talk a lot more Obviously along with your workforce or buyers. It turns an summary grievance into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to take care of error messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept cautiously As well as in entire. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out building assumptions. But deeper during the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into engines like google — read and fully grasp them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or function activated it? These questions can information your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently follow predictable designs, and Discovering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out linked log entries, input values, and up to date variations in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Wisely
Logging is Just about the most effective equipment in the developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, helping you understand what’s going on underneath the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for typical gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the application, Mistake for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure vital messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and important determination factors inside your code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and reliability of the code.
Assume Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers should solution the procedure like a detective solving a mystery. This attitude will help stop working advanced challenges into workable elements and comply with clues logically to uncover the foundation bring about.
Get started by accumulating proof. Think about the symptoms of the problem: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may devoid of leaping to conclusions. Use logs, examination conditions, and person stories to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to on your own: What could be causing this actions? Have any improvements not long ago been manufactured for the codebase? Has this problem occurred right before underneath very similar situation? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled environment. Should you suspect a specific perform or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and Enable the outcome guide you nearer to the truth.
Fork out close notice to modest particulars. Bugs normally conceal while in the least predicted locations—similar to a missing semicolon, an off-by-a person mistake, or a race affliction. Be thorough and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes might cover the real dilemma, just for it to resurface later.
And lastly, preserve notes on Anything you attempted and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Some others comprehend your reasoning.
By wondering like a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Publish Checks
Crafting tests is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Assessments not only assist catch bugs early but in addition serve as a safety Internet that provides you self confidence when building variations towards your codebase. A well-examined application is easier to debug since it permits you to pinpoint specifically in which and when a difficulty happens.
Begin with unit tests, which concentrate on specific features or modules. These tiny, isolated exams can swiftly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you straight away know where by to glimpse, appreciably cutting down enough time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously being preset.
Upcoming, integrate integration tests and close-to-conclude exams into your workflow. These help make sure several areas of your application work jointly easily. They’re especially practical for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. As soon as the check fails persistently, you can target correcting the bug and watch your examination go when The difficulty is settled. This technique makes certain that exactly the same bug doesn’t return Later on.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable course of action—encouraging you catch much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky challenge, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Getting breaks can help you reset your head, cut down irritation, and infrequently see The difficulty from the new point of view.
If you're far too near to the code for way too very long, cognitive exhaustion sets in. You would possibly start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious operate within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then have a 5–ten moment split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it basically brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each bug you come across is much more than simply A short lived setback—It is a chance to improve to be a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better methods like unit testing, code critiques, or logging? The answers click here frequently reveal blind spots in your workflow or understanding and assist you to build stronger coding behavior shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or typical errors—that you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially potent. Whether it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the similar challenge boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders are not those who write fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer thanks to it.
Conclusion
Strengthening your debugging abilities can take time, practice, and persistence — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.